AI with Swift : Introduction
Artificial Intelligence (AI) and machine learning are revolutionizing app development. They enable the creation of intelligent, responsive applications on Apple platforms. “Practical Artificial Intelligence with Swift” is a comprehensive guide. It helps programmers integrate AI into Swift applications.
AI is accessible to all developers now. With the right tools, developers can build features that identify images, make predictions, generate content, and recommend products. Swift, Apple’s powerful programming language, supports AI and machine learning frameworks well.
This book takes a task-based approach. It guides you through building AI-driven features step-by-step. You’ll learn Swift-based AI and machine learning techniques. The book covers tools like Turi Create and Swift for TensorFlow. You’ll gain the knowledge to train and build AI models.
Whether you’re developing for iOS, macOS, tvOS, or watchOS, this book is your go-to resource. AI has transformed how we interact with technology. Swift is a powerful tool for developing AI-driven applications. This book bridges fundamental AI theory and practical application using Swift.
Importance of AI in Modern Technology
AI is not just a buzzword; it’s a transformative force that drives innovation across various industries. AI enhances efficiency, provides insights from vast datasets, and automates repetitive tasks, enabling humans to focus on more complex problems. Understanding AI is crucial for developers looking to stay ahead in the technology landscape.
Why Swift for AI Development?
Swift is renowned for its performance, safety, and expressive syntax, making it an ideal choice for AI development. Its interoperability with Objective-C and robust support for frameworks like Core ML makes Swift a preferred language for developing sophisticated AI applications on Apple platforms.
Who Can Use AI With Swift?
AI and machine learning are becoming increasingly accessible, allowing a wide range of professionals to incorporate these technologies into their applications using Swift. Here are the key groups who can benefit from using AI with Swift:
1. App Developers
App developers can use AI with Swift to create more intelligent and responsive applications for Apple’s platforms, including iOS, macOS, tvOS, and watchOS. By integrating AI, developers can enhance their apps with features such as image recognition, natural language processing, and predictive analytics, leading to a more engaging user experience.
Key Benefits:
- Enhanced app functionality
- Improved user engagement
- Competitive edge in the app market
2. Software Engineers
Software engineers with a background in Swift can leverage AI to solve complex problems and automate tasks. This includes building sophisticated systems for data analysis, automation, and user personalization.
Key Benefits:
- Ability to tackle complex problems
- Streamlined development process
- Efficient automation of repetitive tasks
3. Data Scientists
Data scientists can use Swift to develop AI models and integrate them into applications. While traditionally data scientists might use languages like Python or R, Swift offers powerful tools and frameworks that make it a viable option for AI model deployment in Apple ecosystems.
Key Benefits:
- Seamless integration of AI models into apps
- Access to robust AI frameworks and tools
- Ability to work within the Apple ecosystem
4. Product Managers
Product managers who understand AI can better oversee the development of AI-driven features and products. By knowing the capabilities of AI in Swift, they can make informed decisions about what features to include and how to implement them effectively.
Key Benefits:
- Improved product strategy and planning
- Enhanced feature prioritization
- Better collaboration with development teams
5. Researchers and Academics
Researchers and academics can use AI with Swift to develop prototypes and conduct experiments. Swift’s powerful performance and ease of use make it an excellent choice for exploring new AI techniques and applications.
Key Benefits:
- Efficient development of research prototypes
- Ability to test and validate AI models
- Contribution to the academic body of AI knowledge
6. Entrepreneurs and Startups
Entrepreneurs and startups can leverage AI with Swift to develop innovative products and services. AI-driven features can provide a competitive edge and open up new market opportunities.
Key Benefits:
- Creation of innovative products
- Competitive differentiation
- Access to new market opportunities
7. Educators and Students
Educators and students in computer science and related fields can use AI with Swift to learn about AI and machine learning. Swift’s user-friendly syntax and powerful capabilities make it an excellent language for educational purposes.
Key Benefits:
- Hands-on learning experience with AI
- Understanding of practical AI applications
- Preparation for careers in AI and app development
Fundamental Theory
Understanding Artificial Intelligence
AI refers to the simulation of human intelligence in machines that are programmed to think and learn. It encompasses various subfields such as machine learning, deep learning, neural networks, and natural language processing.
History and Evolution of AI
AI’s journey began in the 1950s with the advent of computers capable of performing tasks that required human intelligence. Over the decades, AI has evolved from rule-based systems to sophisticated models that learn from data, thanks to advancements in computing power and algorithms.
Core Concepts in AI
- Machine Learning: A subset of AI that involves training models on data to make predictions or decisions without being explicitly programmed.
- Deep Learning: A type of machine learning that uses neural networks with many layers to model complex patterns in data.
- Neural Networks: Computational models inspired by the human brain’s structure, used to recognize patterns and make predictions.
- Natural Language Processing (NLP): A field of AI focused on enabling machines to understand and respond to human language.
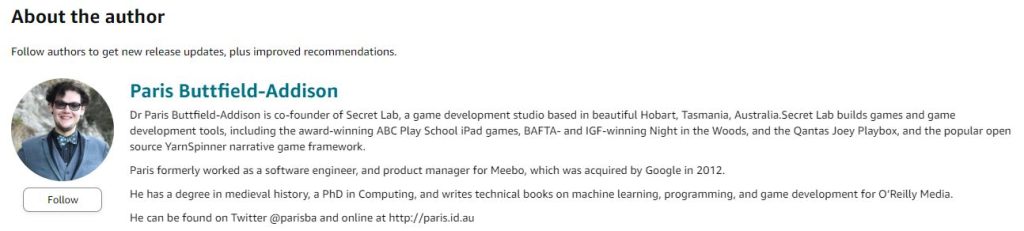
Swift Programming Language
Introduction to Swift
Swift is a modern programming language designed by Apple for iOS, macOS, watchOS, and tvOS app development. It is known for its speed, safety, and expressiveness, making it a favorite among developers.
History of Swift
Swift was introduced by Apple in 2014 as a replacement for Objective-C. It has since undergone numerous updates, becoming one of the most popular programming languages for mobile and desktop application development.
Core Features of Swift
- Syntax and Readability: Swift’s syntax is clean and expressive, making code easy to read and write.
- Performance and Safety: Swift is designed for performance, with safety features that prevent common programming errors.
- Interoperability with Objective-C: Swift can seamlessly interact with Objective-C code, allowing developers to integrate new features into existing projects.
Setting Up the Development Environment
Installing Xcode
Xcode is the integrated development environment (IDE) for Swift. It provides tools for developing, testing, and debugging iOS and macOS applications. Installing Xcode is the first step in setting up your Swift development environment.
Setting Up Swift Playgrounds
Swift Playgrounds is an interactive environment for learning and experimenting with Swift code. It is a great tool for testing small snippets of code and understanding Swift’s syntax and features.
Integrating AI Libraries
Swift supports various AI libraries, including Core ML and TensorFlow. Integrating these libraries into your projects allows you to leverage pre-built models and powerful machine learning capabilities.
Basic AI Concepts with Swift
Data Types and Structures in Swift
Understanding Swift’s data types and structures is fundamental to AI development. Swift offers a variety of data types, including integers, floats, strings, arrays, and dictionaries, which are essential for handling data in AI applications.
Control Flow and Functions
Control flow statements like loops and conditionals, along with functions, are crucial for implementing AI algorithms. Swift’s syntax makes it easy to write and understand these constructs.
Object-Oriented Programming in Swift
Object-oriented programming (OOP) is a paradigm that uses objects and classes to organize code. Swift’s robust support for OOP allows developers to create modular, reusable code, which is essential for large AI projects.
Machine Learning with Swift
Overview of Machine Learning
Machine learning involves training algorithms to learn patterns from data and make predictions. It is a key component of AI and can be implemented using various techniques and algorithms.
Implementing Machine Learning Algorithms in Swift
- Linear Regression: A simple algorithm used for predicting a continuous outcome based on one or more input features.
- Decision Trees: A model that makes decisions based on a series of questions about the input features.
- Clustering: A technique for grouping similar data points together, often used for exploratory data analysis.
Using Swift for TensorFlow
Swift for TensorFlow is a powerful framework that combines the expressiveness of Swift with the capabilities of TensorFlow, allowing developers to build and train complex machine learning models with ease.
Developing Neural Networks
Basics of Neural Networks
Neural networks are a foundational concept in deep learning, consisting of layers of interconnected nodes that process data and learn patterns. Understanding the basics of neural networks is crucial for developing AI applications.
Implementing Neural Networks in Swift
Swift provides tools and libraries for implementing neural networks, including building layers, defining activation functions, and setting up training loops.
Training and Testing Neural Networks
Training a neural network involves feeding it data and adjusting its weights to minimize error. Testing ensures the model performs well on unseen data. Swift’s libraries provide support for both training and testing neural networks.
Natural Language Processing with Swift
Basics of Natural Language Processing
NLP focuses on enabling machines to understand and interact with human language. Tasks include text classification, sentiment analysis, and language translation.
Implementing NLP Tasks in Swift
- Text Classification: Categorizing text into predefined classes, such as spam detection.
- Sentiment Analysis: Determining the sentiment expressed in a piece of text, such as positive or negative reviews.
- Language Translation: Translating text from one language to another using machine translation models.
AI-Driven App Development
Design Principles for AI Apps
Designing AI-driven apps requires careful consideration of user experience, data privacy, and performance. Key principles include simplicity, transparency, and responsiveness.
Developing AI-Driven iOS Apps
Swift, combined with frameworks like Core ML, allows developers to create powerful AI-driven iOS apps. Steps include setting up the project, integrating AI models, and designing the user interface.
Case Study: Building a Chatbot
Building a chatbot involves implementing NLP techniques to understand and respond to user queries. This case study demonstrates how to create a simple chatbot using Swift and Core ML.
Advanced Topics in AI with Swift
In the realm of Artificial Intelligence (AI) and machine learning, there are several advanced topics that can significantly enhance the capabilities of your Swift applications. Understanding and implementing these advanced concepts can set your applications apart by offering sophisticated functionalities and performance optimizations. Here are some key advanced topics in AI with Swift:
1. Neural Networks and Deep Learning
Neural Networks are the backbone of many advanced AI applications. They are particularly useful for tasks such as image and speech recognition, natural language processing, and predictive analytics. Deep learning, a subset of machine learning, involves neural networks with many layers (deep neural networks).
Key Points:
- Convolutional Neural Networks (CNNs): Used for image processing tasks, CNNs can recognize patterns and objects within images, making them ideal for applications like facial recognition and image classification.
- Recurrent Neural Networks (RNNs): Suitable for sequential data, RNNs are commonly used in natural language processing, time series prediction, and speech recognition.
- Training Deep Neural Networks: Tools like TensorFlow and Turi Create can be used in Swift to build and train deep neural networks.
2. Natural Language Processing (NLP)
Natural Language Processing enables computers to understand, interpret, and generate human language. This is essential for applications that involve text analysis, translation, sentiment analysis, and conversational agents (chatbots).
Key Points:
- Text Classification: Categorizing text into predefined categories using models such as Naive Bayes, SVM, or deep learning models.
- Sentiment Analysis: Determining the sentiment expressed in a piece of text, which can be useful for social media monitoring, customer feedback analysis, etc.
- Language Translation: Translating text from one language to another using sequence-to-sequence models.
- Tools: Swift packages like NaturalLanguage (by Apple) provide powerful tools for NLP tasks.
3. Reinforcement Learning
Reinforcement Learning (RL) is a type of machine learning where an agent learns to make decisions by performing actions in an environment to maximize cumulative rewards.
Key Points:
- Q-Learning and Deep Q-Networks (DQN): Basic algorithms used in reinforcement learning for decision-making.
- Applications: RL can be applied in game development, robotics, autonomous systems, and more.
- Frameworks: Integrating RL libraries and frameworks with Swift to develop and train RL models.
4. Transfer Learning
Transfer Learning involves taking a pre-trained model and fine-tuning it on a new, related task. This approach is beneficial when you have limited data for the new task but access to a large dataset for a similar task.
Key Points:
- Model Fine-Tuning: Adjusting a pre-trained model’s weights to adapt it to the new task.
- Applications: Image recognition, NLP, and any domain where data is limited but related datasets are available.
- Tools: Utilizing pre-trained models from TensorFlow Hub or Turi Create in Swift projects.
5. Generative Adversarial Networks (GANs)
Generative Adversarial Networks are used to generate new, synthetic data that resembles real data. They consist of two neural networks: a generator and a discriminator.
Key Points:
- Applications: GANs are used in image generation, video synthesis, and creating realistic data for training other models.
- Training GANs: Involves a challenging process of training both the generator and discriminator networks to improve their performance.
- Frameworks: Using Swift for TensorFlow to implement and train GANs.
6. Optimization Techniques
Optimization Techniques are critical for improving the performance and efficiency of AI models.
Key Points:
- Gradient Descent and Its Variants: Including Stochastic Gradient Descent (SGD), Adam, and RMSprop for optimizing neural network training.
- Hyperparameter Tuning: Techniques like grid search, random search, and Bayesian optimization to find the best hyperparameters for your models.
- Model Compression: Techniques like pruning, quantization, and knowledge distillation to reduce model size and increase inference speed.
7. Explainable AI (XAI)
Explainable AI aims to make AI models more interpretable and understandable to humans.
Key Points:
- Model Interpretability: Techniques to explain the predictions of complex models, such as LIME and SHAP.
- Ethics and Transparency: Ensuring AI models are transparent and their decisions can be explained to stakeholders.
- Tools: Libraries and frameworks that support explainability features, integrated with Swift applications.
8. Real-Time AI
Real-Time AI involves deploying AI models that can process data and make decisions in real time.
Key Points:
- Low-Latency Inference: Techniques to reduce inference time, such as model optimization and hardware acceleration.
- Edge Computing: Deploying AI models on edge devices for real-time processing without relying on cloud infrastructure.
- Applications: Real-time AI is crucial in applications like autonomous driving, real-time analytics, and interactive user experiences.

Product details
ASIN : B07ZHPPVK3
Publisher : O’Reilly Media; 1st edition (September 3, 2019)
Publication date : September 3, 2019
Language : English
File size : 50336 KB
Simultaneous device usage : Unlimited
Text-to-Speech : Enabled
Screen Reader : Supported
Enhanced typesetting : Enabled
X-Ray : Not Enabled
Word Wise : Not Enabled
Sticky notes : On Kindle Scribe
Print length : 528 pages
Best Sellers Rank: #853,167 in Kindle Store (See Top 100 in Kindle Store)
47 in Apple Developing & Programming
58 in Swift Programming Language
89 in Apple OS & iOS
Customer Reviews: 4.5 4.5 out of 5 s
Price
AI with Swift: Pros and Cons
Incorporating Artificial Intelligence (AI) with Swift offers a range of advantages, but there are also some challenges to be aware of. Here are the key pros and cons of using AI with Swift:
Pros
- Integration with Apple Ecosystem
- Seamless Integration: Swift is designed for Apple platforms, allowing for seamless integration of AI features in iOS, macOS, tvOS, and watchOS applications.
- Unified Development Environment: Developers can use Xcode, Apple’s integrated development environment (IDE), to build and test their applications efficiently.
- Performance
- High Performance: Swift is known for its speed and performance. AI applications, especially those requiring real-time processing, benefit from Swift’s efficiency.
- Memory Management: Swift’s memory management features, like Automatic Reference Counting (ARC), help in optimizing performance and preventing memory leaks.
- User-Friendly Syntax
- Readable Code: Swift’s syntax is clean and easy to read, making it more approachable for developers new to AI or those transitioning from other languages.
- Faster Development: The concise syntax allows for faster development and easier maintenance of AI models and applications.
- Powerful Frameworks
- Core ML: Apple’s Core ML framework simplifies the integration of machine learning models into Swift applications. It supports various model formats and offers easy deployment.
- Turi Create and Swift for TensorFlow: These tools provide additional capabilities for training and deploying AI models, making Swift a versatile option for AI development.
- Strong Community and Support
- Active Community: Swift has a robust and active developer community, which means abundant resources, tutorials, and forums for troubleshooting and learning.
- Official Documentation: Apple provides extensive and well-maintained documentation for Swift and its related frameworks.
- Security
- Safety Features: Swift includes several safety features that reduce the likelihood of common programming errors, such as null pointer dereferencing, which can be particularly beneficial when developing complex AI algorithms.
Cons
- Limited Third-Party Libraries
- Fewer Libraries: Compared to Python, Swift has fewer third-party libraries specifically for AI and machine learning, which can limit the available tools and functionalities.
- Dependency Management: Managing dependencies and integrating third-party AI libraries can be more challenging in Swift than in languages with more established ecosystems like Python.
- Learning Curve
- New Paradigms: For developers coming from non-Swift backgrounds, especially those familiar with languages like Python or Java, there can be a learning curve to adapt to Swift’s syntax and paradigms.
- Swift-Specific Knowledge: Understanding Swift’s specific frameworks and tools for AI might require additional learning and adaptation.
- Platform Limitation
- Apple-Centric: Swift is primarily designed for Apple platforms, which can be a limitation if cross-platform compatibility is a requirement for your AI application.
- Deployment Restrictions: Deploying AI applications developed in Swift on non-Apple platforms might require additional effort or be impractical.
- Tooling Maturity
- Emerging Tools: Some AI and machine learning tools for Swift, like Swift for TensorFlow, are still emerging and might not be as mature or feature-complete as their counterparts in other languages.
- Rapid Evolution: Swift and its AI tools are evolving quickly, which can lead to compatibility issues or require frequent updates to keep up with the latest features and best practices.
- Community Size
- Smaller AI Community: The AI community around Swift is smaller compared to Python, which means fewer shared resources, less community-driven support, and fewer specialized AI conferences or meetups.
Conclusion
Mastering these advanced topics in AI with Swift can significantly elevate the capabilities of your applications. By integrating neural networks, NLP, reinforcement learning, transfer learning, GANs, optimization techniques, explainable AI, and real-time AI, you can develop sophisticated, high-performance applications that leverage the full potential of artificial intelligence. This comprehensive approach will not only enhance user experience but also position your apps at the forefront of technological innovation.
>>As An Early Bird Click Here To Get AI with Swift<<
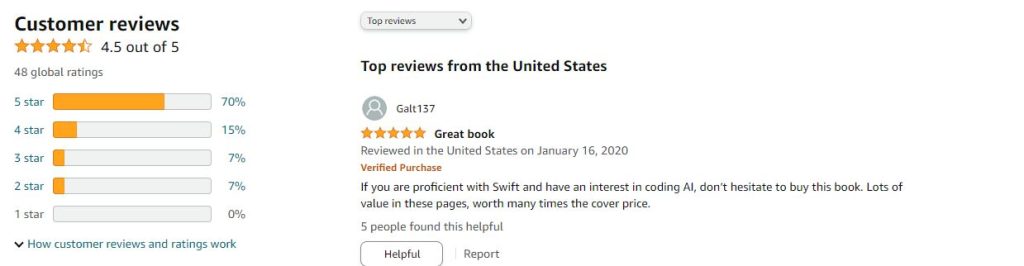
Frequently Asked Questions
1. What is the focus of “Practical Artificial Intelligence with Swift”?
Answer: The book focuses on integrating Artificial Intelligence (AI) and machine learning into applications developed using Swift. It covers fundamental AI theory and provides practical guidance on implementing AI-driven features in apps for iOS, macOS, tvOS, and watchOS.
2. Do I need a background in data science to benefit from this book?
Answer: No, you don’t need a background in data science. The book is designed for programmers and developers of all levels. It explains AI concepts in an accessible manner and guides you through the process of implementing Artificial Intelligence features using Swift.
3. What are some AI features I can build using Swift?
Answer: Using Swift, you can build AI features such as image identification, predictions, content generation, product recommendations, text classification, sentiment analysis, and language translation.
4. Why should I use Swift for Artificial Intelligence development?
Answer: Swift is a powerful and intuitive programming language with strong support for AI and machine learning frameworks. Its performance, safety features, and interoperability with Objective-C make it an ideal choice for developing sophisticated AI applications on Apple platforms.
5. What tools and frameworks are covered in the book?
Answer: The book covers tools and frameworks such as Apple’s Core ML, Python-powered Turi Create, and Google’s Swift for TensorFlow. These tools help you train and build AI models effectively.
6. How does the book approach teaching AI with Swift?
Answer: The book takes a task-based approach, providing step-by-step guidance on building AI-driven features. It includes practical examples and real-world case studies to illustrate how to implement and use various AI techniques in Swift.
7. Can I use this book to develop AI apps for multiple Apple platforms?
Answer: Yes, the book is applicable to developing AI-driven applications across multiple Apple platforms, including iOS, macOS, tvOS, and watchOS.
8. What kind of prior knowledge do I need to get started?
Answer: Basic knowledge of Swift programming is recommended, but the book is designed to be accessible to developers at different skill levels. It starts with fundamental concepts and gradually introduces more complex topics.
9. How does AI enhance app functionality?
Answer: AI enhances app functionality by enabling features like intelligent data analysis, personalized user experiences, automation of repetitive tasks, and improved decision-making based on predictive models.
10. What are some practical applications of AI covered in the book?
Answer: The book covers practical applications of AI in various fields, including healthcare (diagnostics and patient monitoring), finance (fraud detection and risk assessment), and entertainment (recommendation engines and content creation).
11. How do I test and debug AI models in Swift?
Answer: The book provides strategies for testing and debugging AI models in Swift. It covers unit testing, model validation, and performance optimization to ensure your AI features work correctly and efficiently.
12. What are the future prospects of AI development with Swift?
Answer: The future of AI development with Swift looks promising with ongoing advancements in AI frameworks and tools. The book discusses emerging trends and future developments in AI and how Swift continues to play a crucial role in this evolving field.
13. Where can I find additional resources for learning AI with Swift?
Answer: The book includes a section on additional resources, recommending books, online courses, and websites to further enhance your knowledge and skills in Artificial Intelligence development with Swift.
14. How can AI-driven features add value to my apps?
Answer: AI-driven features can add value by improving user engagement, providing personalized experiences, automating complex tasks, and offering predictive insights that enhance the overall functionality and user satisfaction of your apps.
15. Is this book suitable for someone new to AI and machine learning?
Answer: Yes, the book is suitable for beginners. It introduces AI and machine learning concepts in a straightforward manner and provides practical examples to help you understand and implement these technologies in your Swift applications.
Learn About A I Agents Exposed Review
Affiliate Disclaimer:
I will receive affiliate commissions for reviews and promotions on this website. Always provide my honest assessment, relevant experience, and sincere opinion about genuine goods and services. I want to be as helpful as possible to help you choose what to buy, your opinion, and your perspective. I am prepare to provide you with my particulars and insights, facilitating your ongoing investment at an appropriate level. However, before any acquisition , ensure thorough verification of the outcomes and metrics . By clicking on links or purchasing items offered on this website, you can financially support me through affiliate commissions. “It’s important for you to understand that I receive compensation upon your purchase.”